- Регистрация
- 27 Авг 2018
- Сообщения
- 37,369
- Реакции
- 524,677
- Тема Автор Вы автор данного материала? |
- #1
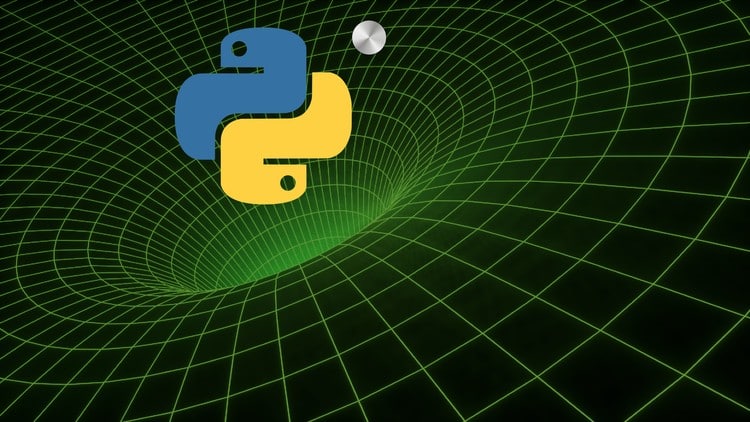
- An in-depth look at variables, memory, namespaces and scopes
- A deep dive into Python's memory management and optimizations
- In-depth understanding and advanced usage of Python's numerical data types (Booleans, Integers, Floats, Decimals, Fractions, Complex Numbers)
- Advanced Boolean expressions and operators
- Advanced usage of callables including functions, lambdas and closures
- Functional programming techniques such as map, reduce, filter, and partials
- Create advanced decorators, including parametrized decorators, class decorators, and decorator classes
- Advanced decorator applications such as memoization and single dispatch generic functions
- Use and understand Python's complex Module and Package system
- Idiomatic Python and best practices
- Understand Python's compile-time and run-time and how this affects your code
- Avoid common pitfalls
- Basic introductory knowledge of Python programming (variables, conditional statements, loops, functions, lists, tuples, dictionaries, classes).
- You will need Python 3.6 or above, and a development environment of your choice (command line, PyCharm, Jupyter, etc.)
Hello!
This is Part 1 of a series of courses intended to dive into the inner mechanics and more complicated aspects of Python 3.
This is not a beginner course - if you've been coding Python for a week or a couple of months, you probably should keep writing Python for a bit more before tackling this series.
On the other hand, if you're now starting to ask yourself questions like:
- I wonder how this works?
- is there another way of doing this?
- what's a closure? is that the same as a lambda?
- I know how to use a decorator someone else wrote, but how does it work? Can I write my own?
- why isn't this boolean expression returning a boolean value?
- what does an import actually do, and why am I getting side effects?
- and similar types of question...
Please make sure you review the pre-requisites for this course (below) - although I give a brief refresh of basic concepts at the beginning of the course, those are concepts you should already be very comfortable with as you being this course.
In this course series, I will give you a much more fundamental and deeper understanding of the Python language and the standard library.
Python is called a "batteries-included" language for good reason - there is a ton of functionality in base Python that remains to be explored and studied.
So this course is not about explaining my favorite 3rd party libraries - it's about Python, as a language, and the standard library.
In particular this course is based on the canonical CPython. You will also need Jupyter Notebooks to view the downloadable fully-annotated Python notebooks.
It's about helping you explore Python and answer questions you are asking yourself as you develop more and more with the language.
In Python 3: Deep Dive (Part 1) we will take a much closer look at:
- Variables - in particular that they are just symbols pointing to objects in memory
- Namespaces and scope
- Python's numeric types
- Python boolean type - there's more to a simple or statement than you might think!
- Run-time vs compile-time and how that affects function defaults, decorators, importing modules, etc
- Functions in general (including lambdas)
- Functional programming techniques (such as map, reduce, filter, zip, etc)
- Closures
- Decorators
- Imports, modules and packages
- Tuples as data structures
- Named tuples
Course Prerequisites
This is an intermediate to advanced Python course.
To have the full benefit of this course you should be comfortable with the basic Python language including:
- variables and simple types such as str , bool , int and float types
- for and while loops
- if...else... statements
- using simple lists , tuples , dictionaries and sets
- defining functions (using the def statement)
- writing simple classes using the class keyword and the __init__ method, writing instance methods, creating basic properties using @property decorators
- importing modules from the standard library (e.g. import math)
- have Python 3.6 (or higher) installed on your system
- be able to write and run Python programs using either:
- the command line, or
- a favorite IDE (such as PyCharm),
- have Jupyter Notebooks installed (which I use throughout this course so as to provide you fully annotated Python code samples)
- Anyone with a basic understanding of Python that wants to take it to the next level and get a really deep understanding of the Python language and its data structures.
- Anyone preparing for an in-depth Python technical interview.
DOWNLOAD: